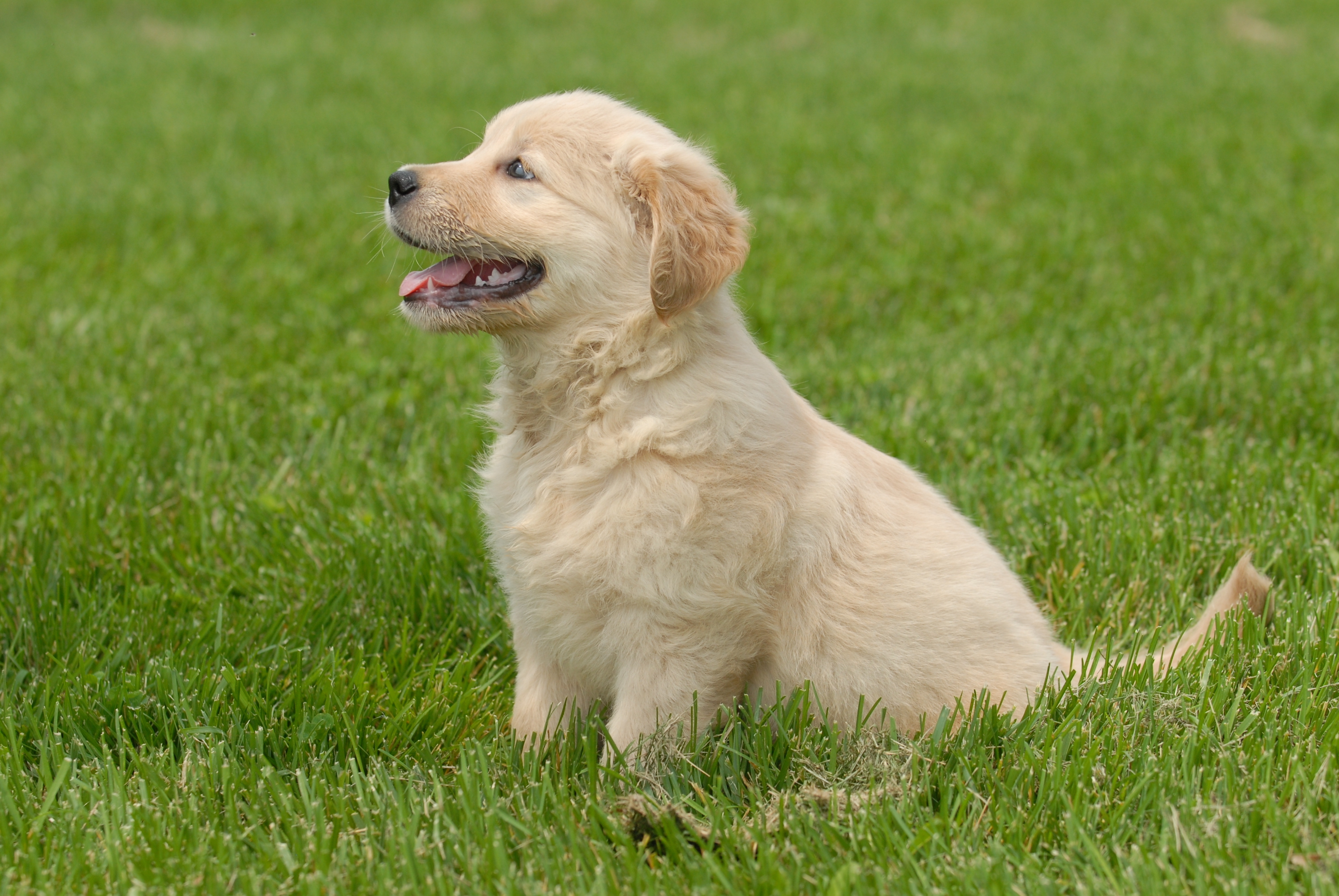
Cashew
Cashew is a playful golden retriever puppy, full of energy and affection, looking for a loving owner to share adventures with.
Cashew is a playful golden retriever puppy, full of energy and affection, looking for a loving owner to share adventures with.
npm i clsx tailwind-merge framer-motion
import clsx, { ClassValue } from "clsx";
import { twMerge } from "tailwind-merge";
export function cn(...inputs: ClassValue[]) {
return twMerge(clsx(inputs));
}
interface RevealCard2Props extends React.HTMLAttributes<HTMLDivElement> {
src: string;
alt: string;
}
export const RevealCard2: React.FC<RevealCard2Props> = ({ src, alt, children, className, ...props }) => {
return (
<div>
<article className={cn(styles.card, className)}>
<img className={cn(styles.card__background)} src={src} alt={alt} width="1920" height="2193" />
<div className={cn(styles.card__content, styles.flow)}>
<div className={cn(styles.card__content_container, styles.flow)} {...props}>
{children}
</div>
</div>
</article>
</div>
);
};
interface RevealCard2TitleProps extends React.HTMLAttributes<HTMLDivElement> {}
export const RevealCard2Title: React.FC<RevealCard2TitleProps> = ({ children, className, ...props }) => {
return (
<h2 className={cn(styles.card__title, className)} {...props}>
{children}
</h2>
);
};
interface RevealCard2DescriptionProps extends React.HTMLAttributes<HTMLDivElement> {}
export const RevealCard2Description: React.FC<RevealCard2DescriptionProps> = ({ children, className, ...props }) => {
return (
<p className={cn(styles.card__description, className)} {...props}>
{children}
</p>
);
};
interface RevealCard2ButtonProps extends React.ButtonHTMLAttributes<HTMLButtonElement> {
onClick?: () => void;
}
export const RevealCard2Button: React.FC<RevealCard2ButtonProps> = ({ children, className, onClick, ...props }) => {
return (
<button className={cn(styles.card__button, className)} onClick={onClick} {...props}>
{children}
</button>
);
};